Collection of Commands
-----Kubectl commands with Powershell scripts-----
kubectl apply -f path/to/yaml/file/test.yaml - Apply yaml file and deploy k8s resource.
Kubernetes cleanup - deleting all configmaps, secrets, jobs and pods
kubectl delete -n default configmap --all
kubectl delete -n default secret --all
kubectl delete -n default jobs --all
kubectl delete -n default pods --all
Deleting resources - jobs and pods that start with some prefix
# List all Jobs and filter by those starting with "imputcs"
$jobs = kubectl get jobs --no-headers | Where-Object { $_.Split()[0] -like "imputcs*" }
# Loop through the filtered Jobs and delete each one
foreach ($job in $jobs) {
$jobName = $job.Split()[0]
Write-Host "Deleting Job: $jobName"
kubectl delete job $jobName
}
# List all Pods and filter by those starting with "imputcs"
$pods = kubectl get pods --no-headers | Where-Object { $_.Split()[0] -like "imputcs*" }
# Loop through the filtered Pods and delete each one
foreach ($pod in $pods) {
$podName = $pod.Split()[0]
Write-Host "Deleting Pod: $podName"
kubectl delete pod $podName
}
-----Linux-----
kf -kh . - Displays information about total space and available space on a file system. Flag -k displays statistics in units of 1024-byte blocks.
ps -p YourProcessID -o lstart= - See when a process started running. Another option (ps -f |grep pid)
ps aux | grep yourUserName - List all processes currently running that are associated with a user.
date - Get current date and time.
kill -QUIT YourProcessID - Kill process and get the thread dump.
rmdir directoryName - Remove empty directory
rm -r directoryName - Remove directory and all it's contents
ps -o thcount YourProcessID - To get the number of threads for a given pid.
cd - or cd .. - Go back one directory.
cd ~ - Go to home directory.
clear or ctrl + l - Clear the screen.
history - History of commands used.
reset - Resets the shell.
ctrl + u - Delete a line typed into the shell.
ctrl + a - Go to start of line.
ctrl + e - Go to end of line.
command1;command2 - Run multiple commands - Chaining commands. Better than 'command1 && command2' (failure of command 1 here will not execute command 2)
someRandomCommand | column -t - Produce the output in the form of a table if supported.
-----htop: An interactive process viewer for Linux-----
- Start htop using the following command- htop.
- Enter setup menu by pressing F2.
- From leftmost column choose "Columns".
- From rightmost column choose the column to be added to main monitoring output. NLWP is for Number of light weight processes using threads.
- Press F10 to finish setup.
-----Git-----
git branch branchName - Log info on commitCreate a new branch off of the current branch.
git checkout -b branchName - Create new branch and switch to it
git checkout branchName - Switch to an existing branch.
git log - Log info on commits.
git checkout SHA - Checkout a specific commit. SHA can be acquired through git log.
-----JVM Options-----
-XX:CompileCommand="exclude,java/lang/Object.wait" - To prevent Object.wait from being JIT-compiled (and thus making wait info always available)
-Xmx_g - Selects the maximum memory sizes available to the JVM. These values are used for the JVM heap, which reserves memory. Something like -Xmx16g [Remember to replace the _]
-d64 - For 64-bit machines only. This options should be specified when a large JVM heap is required (greater than 4 Gbytes) and the architecture is 64-bit.
-XX:NewSize=512M - In heavy throughput environments, you should consider using this option to increase the size of the JVM young generation. By default, the young generation is quite small, and high throughput scenarios can result in a large amount of generated garbage. This garbage collection, in turn, causes the JVM to inadvertently promote short-lived objects into the old generation.
-----Other helpful Linux commands-----
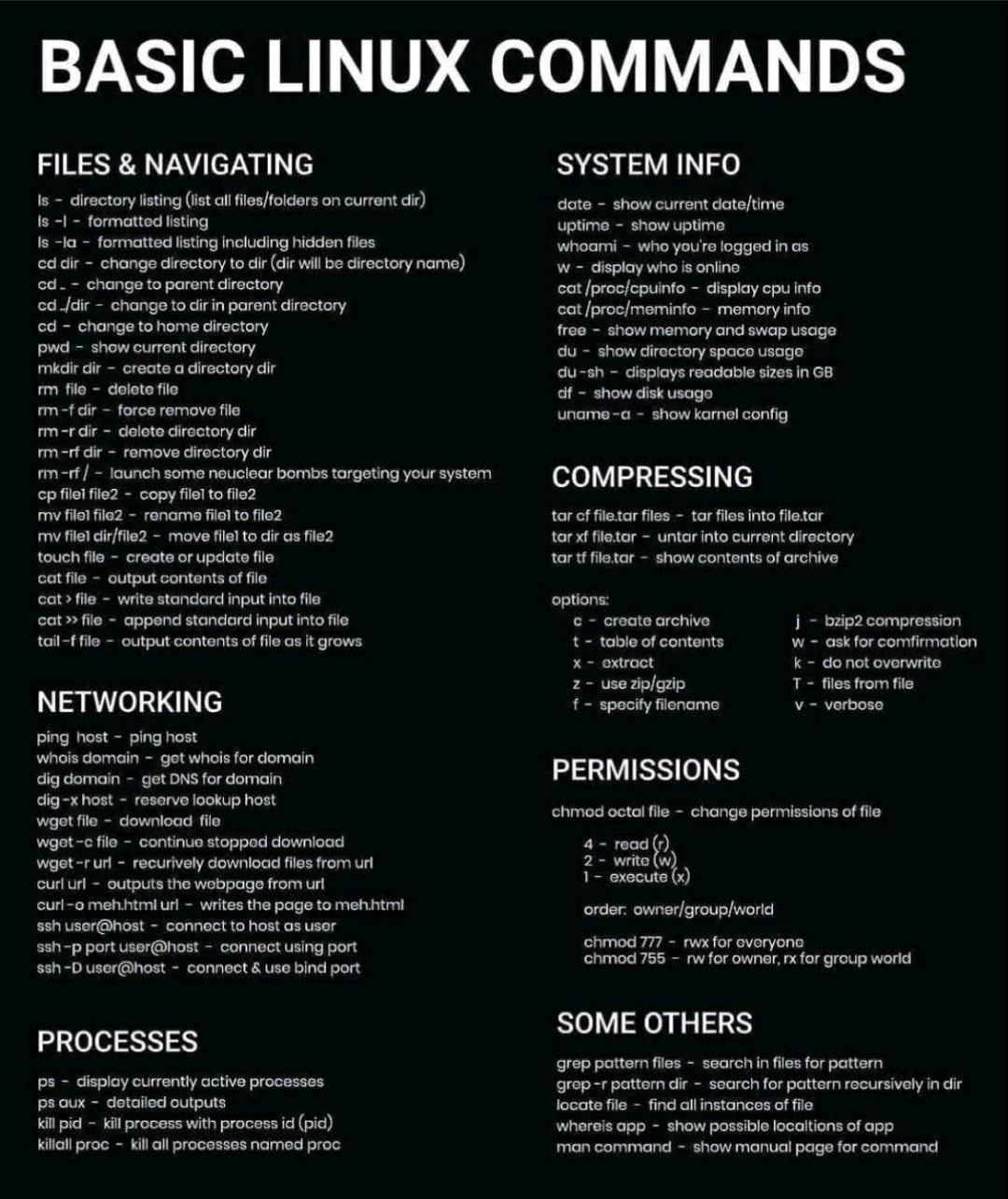